Probably anyone who uses Oracle Forms Builder, at some point found himself with a certain problem that is very hard to fix using Oracle Forms Builder only, although it gives prety much everything you need to build a database application.
On the other hand , large number of those who managed to solve the problem weren’t even aware of a fact that they were able to solve that ’large’ and unusual problem by using Java beans, which can be used in Oracle Forms Builder.
Not to mention that many developers don’t even know about this functionality of Forms Builder that enables us to use Java code in our Oracle Forms application, and those who do know about it don’t know HOW to do it!
What I now plan to do is to show you how you can create and use some simple java bean in your Oracle Forms application.
These examples are far from usefull and complex but it will help you understand how to make a java bean and then you can create very usefull and complexe modules using almost complete power of Java...
I use Oracle 10g , and Developer suite 10g , and that means that we must use 1.4 version of java while building our bean.
Let’s open Eclipse and create new Java Project. Let’s call it „MyBean“ , and let’s create one Java class called „FirstBean“. For now, we have something like this:
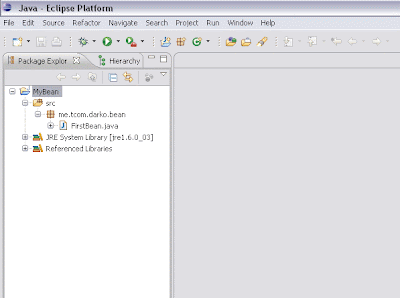
The easiest way of creating a java bean that we can use in oracle forms builder, is to extend Vbean class. Vbean is located in frmall.jar. You can find it in %ORACLE_HOME%\forms\java on windows. ( for example, I have it on location C:\oracle\DevSuite\forms\java )
In order to use it, open project -> properties in eclipse , and under „java build path“ click on the Libraries tab , and then select frmall.jar by clicking on the „Add external jars“ button.
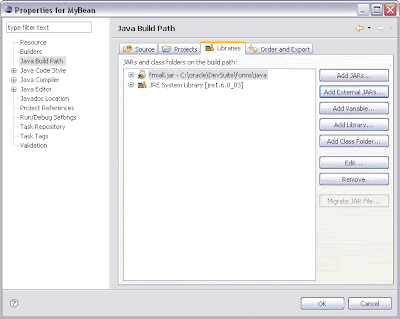
I mentioned before that we must use 1.4 compiler compliance level , so select it from drop down list, under „Java compiler“.
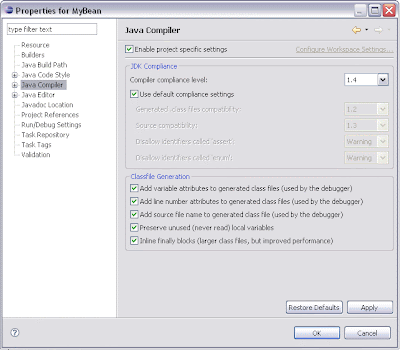
Click apply .
I will make a bean that we can use to:
- Get our own IP address
- Switch letters case of some string to upper case
- and to show input dialog for a user to enter some string and retrieve that string.
These examples are more than trivial , but they will help you understand a logic of building java beans that you can use in oracle forms builder.
You will soon see that we communicate with java beans by setting and getting some custom properties. all this is done by the use of the getPropery and setPropety methods defined in the Iview interface. Each property is created and stored within the oracle.forms.properties.ID class.
Let’s make 5 of it:

We will use I_TEXT to set an input dialog text, IP to retrieve ip address , SET_STRING to set a string for which we change letters case , SHOW_INPUT to say a java bean that we want to show input dialog, and UPPER_CASE to say a java bean that we want to retrieve (upper cased) string that we sent earlier.
So, the only two things we need to set before getting the result from java bean are I_TEXT ( text to be shown on input dialog) and SET_STRING ( the string that is about to be upper cased ).
In order to set it, we must use setter setProperty() :
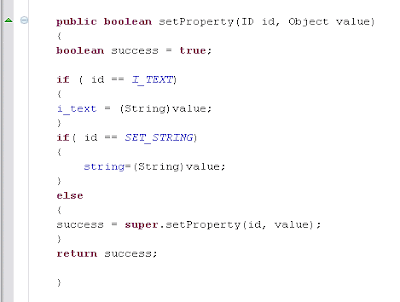
and later when we call SET_CUSTOM_PROPERTY in PL/SQL we will send it’s value.
If we have a BEAN_AREA in oracle forms called BLOCK4.BEAN_AREA11 , and want to set I_TEXT ( input dialog text ) to be : „Please insert a value:“ , we would call
Set_Custom_Property('BLOCK4.BEAN_AREA11',1, 'I_TEXT', ’Please insert a value:’);
The other three ID classes are used to get a value ( remember? : IP , upper case string, and input dialog entry )
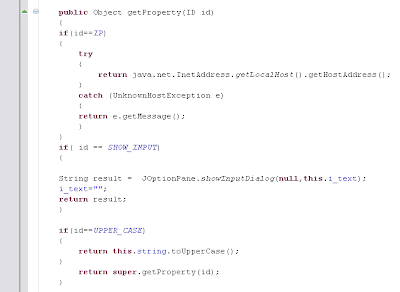
If we have a BEAN_AREA in oracle forms called BLOCK4.BEAN_AREA11 , and want to get and show user’s entry from the input dialog into the :BLOCK4.TEXT_ITEM10 , we would get it by calling:
:BLOCK4.TEXT_ITEM10:=GET_CUSTOM_PROPERTY('BLOCK4.BEAN_AREA11',1,'SHOW_INPUT');
In eclipse, right click on MyBean project, and select „Export“. Follow the wizzard .
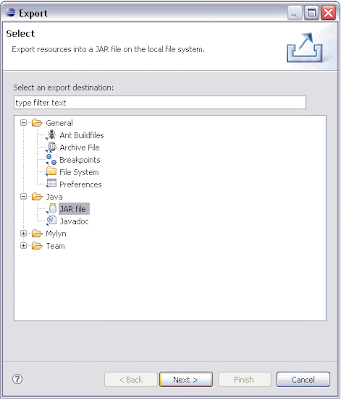
Copy the .jar file you exported into %ORACLE_HOME%\forms\java folder ( in the same folder where frmall.jar is located ).
Update %ORACLE_HOME%\forms\server\formsweb.cfg with „mybean.jar“ entry:
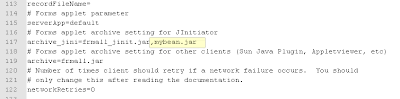
in the registry editor update FORMS_BUILDER_CLASSPATH variable :
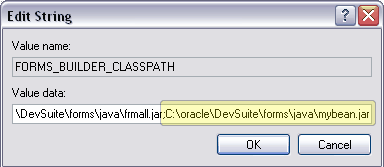
Start OC4J instance ( START -> Oracle developer suite -> Forms Developer -> Srart OC4J instance ) .
Start Forms builder.
me.tcom.darko.bean.FirstBean
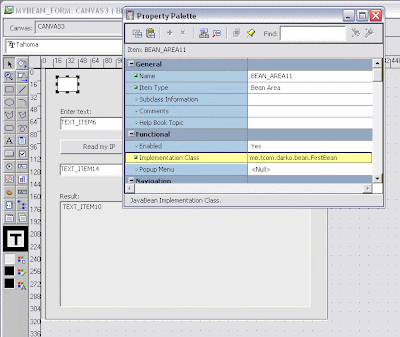
and start using it.
(notice: we are not setting anything in order to get this result )
:BLOCK4.TEXT_ITEM10:=GET_CUSTOM_PROPERTY('BLOCK4.BEAN_AREA11',1,'UPPER_CASE');
(notice: in order to get upper cased string, we need to set it first )
and to show input dialog to user , and retrieve user’s entry:
:BLOCK4.TEXT_ITEM10:=GET_CUSTOM_PROPERTY('BLOCK4.BEAN_AREA11',1,'SHOW_INPUT');
( notice: again, we need to set I_TEXT first in order to show input dialog )
Java beans in oracle forms builder can really be powerful. For many things that you cannot do in Forms builder there is JAVA.
For example you can make java swing application, integrate it into java bean and call it from within oracle forms and many more…
Also, you can register events in oracle forms builder of that swing application and use it , and many more, but some other time about that…
11 comments:
For Implementation Class, I know FirstBean is the name of the java class but where do you get me.tcom.darko.bean. from?
I did't quite understand you...
It's just the name of the package that FirstBean class is located in...
Hi;
Thanks for your post.
I have generated my jar file and signed it.It works on my computer.
But after copying my jar to the application server and want to open it through web; it doesn't work.There are errors on java console:
*** VBean null PropertyManager for id = FOREGROUND
*** VBean Got FOREGROUND = null
*** VBean null PropertyManager for id = BACKGROUND
*** VBean Got BACKGROUND = null
*** VBean Setting beanName to oracle.forms.fd.mypackage.JavaBean
*** VBean Failed to instantiate class: oracle.forms.fd.mypackage.JavaBean java.lang.ClassNotFoundException: oracle.forms.fd.mypackage.JavaBean
*** VBean Setting debugMode to ALL
Do you know about this?
I really can tell just by looking these errors...
It looks to me like your java classpath on the server is not set right...?
What OS is OAS installed on?
Do you have the same version of your Oracle application server on your computer and on the server?
Did you set archive_jini variable in the formsweb.cfg?
It could be a lot of things...
Unfortunately, last time I developed some JARs for Oracle forms application was more than a year ago, at work.
And just developed...
Some other people deployed my .jar to application server and set all the AS parameters...
I don't remember they had any problems deploying it...
Hello Darko,
Thanks for your post. I have a problem to download the zip file with fmb and java source code because probably it's not available anymore. If you still have it I would appreciate if you can send me by email to alexander.reichman@gmail.com
Thanks
Hi, Alexander!
Yes, I noticed this problem before, but for some reason I'm not able to edit this post... (???)
Here are the correct links:
FirstBean.java :
http://www.kdarko.users.t-com.me/download/FirstBean.java
and here is the .zip file:
http://www.kdarko.users.t-com.me/download/mybean.zip
Thanks a lot Darko!
I found the information shared on this blog very helpful and very useful which explains step-by-step process for creating the JavaBean
Hi Can I pass my parameters from java bean ( set as post )and call a servlet.
simply sue, can you explain what exactly would you like to do?
Hello, we cannot download your zip files or java files????
Can u pls rectify this.
Hello,
The links to the source files don't work again, can you please upload them?
Thank you.
Radu
Post a Comment